Haze
A bespoke, from-scratch, full-stack toolchain for my new processor architecture, haze.
github.com/connorjlink/hazeCompiler
Atop a custom recursive-descent parser, compiler implements my own haze compiled programming language with custom backends and linkers for both haze and x86_64 processors.
Displayed below are a sample program compilation input and output.
.include "std.hz"
function byte main()
{
asm
{
.define TEST = 1
.org &$8000
copy r0, #TEST
copy r1, #TEST
iadd r0, r1 ; 1 + 1
}
byte foo = (2 * 3) + 1; // 7
return foo;
}
; Generated by the haze optimizer
; @ 8/14/2024 23:11:02
.org &$8000
main:
copy r0, #7
push r0
exit
Interpreter
Sharing syntax with the haze compiler, interpreter can hook the geo runtime as an intepreted scripting language using custom MQTT-based IPC or execute freestanding.
Shown below are both a standalone "unhook" and geo-interfacing script.
.include "std.hzi"
.define A = 1
.define B = 2
.define C = 3
.macro MUL = (x, y):
{
[x] * [y]
}
function nvr main()
{
// no MQTT hook install
.unhook
byte foo = $MUL(A, B) + C;
print(foo * 2); // output: 10
}
.include "std.hzi"
.define RANGE = 1
.macro X = (a):
{
a[0]
}
intrinsic sky_color = [.5, .5, .5];
intrinsic mouse_sensitivity = 50;
intrinsic fov = 90;
function nvr main(float ΔT)
{
if ($X(sky_color) < RANGE)
$X(sky_color) += ΔT;
else if ($X(sky_color) > RANGE)
$X(sky_color) -= ΔT;
}
Assembler
Internally utilizing the powerful haze frontend, assembler transforms custom assembly language source code into haze machine code
Emulator
Simulating haze machine code program execution at several KHz, emulator eases smoke-testing with live data watches and on-the-fly disassembly.
Language Server
A fully LSP-compliant language server implementation, haze-ls offers contextual autocomplete and custom syntax highlighting for the haze language in VSCode.
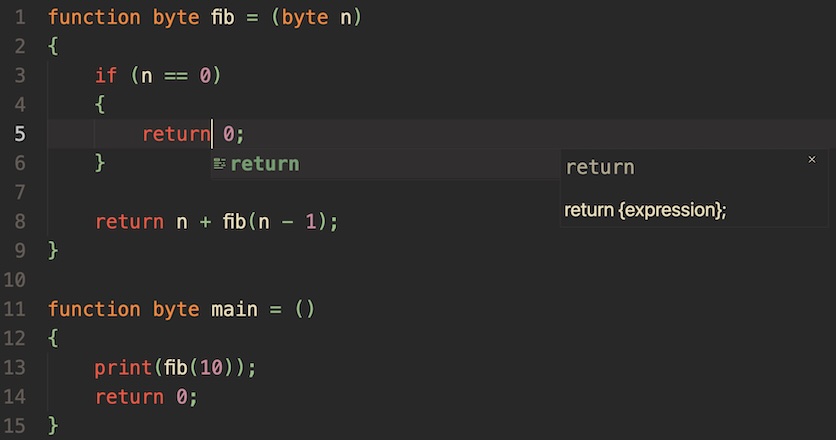
Inspector
An efficiency-first MQTT traffic monitoring client, inspector offers robust send/receive capabilities and high-level automation in a user-friendly WPF-based window.
github.com/connorjlink/inspector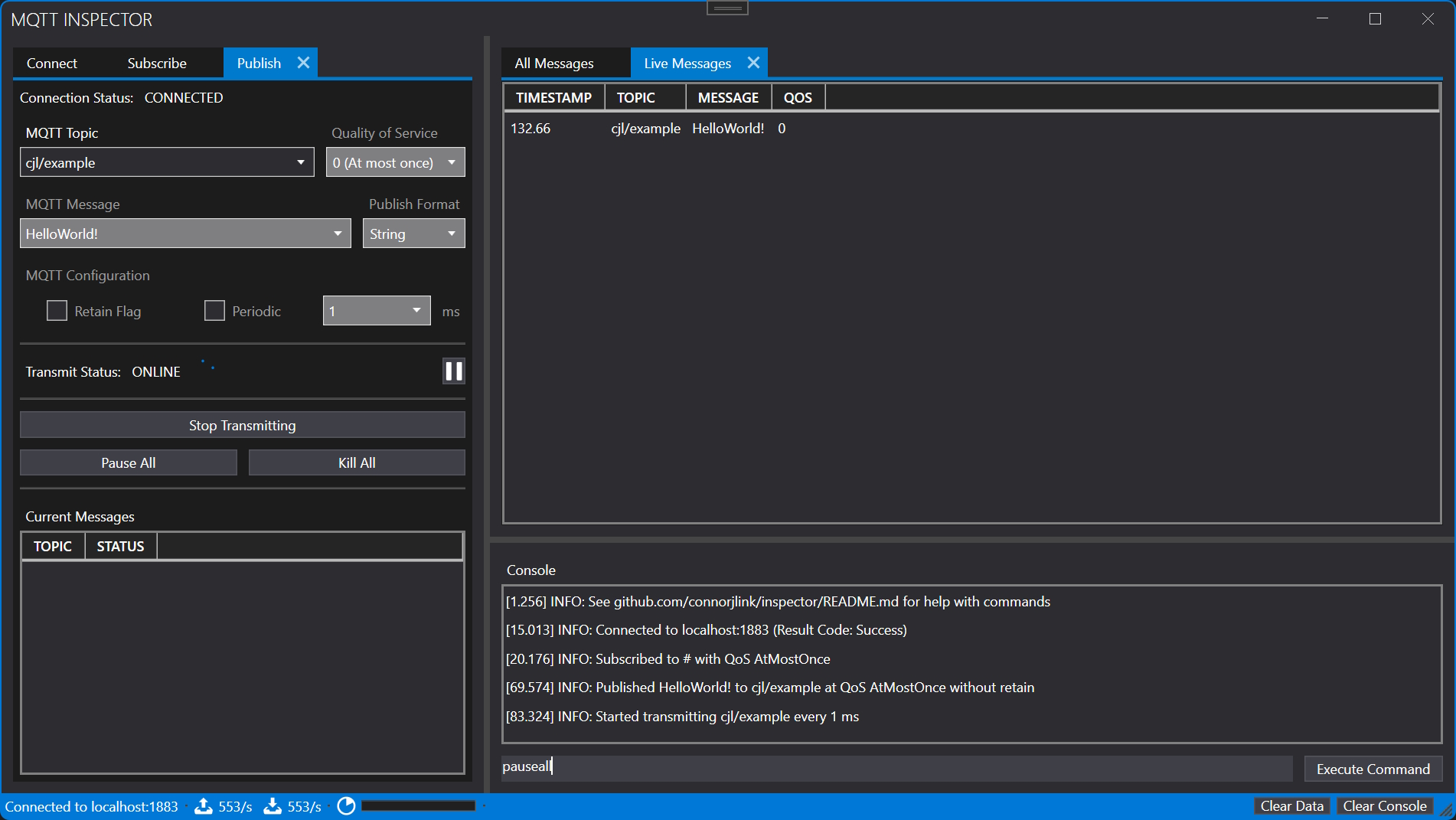
3-D Rendering
Voxelization
A modern-OpenGL–based voxel rendering program, geo utilizes geometry pre-generation, vertex batching, and indexed instancing for remarkable efficiency.
github.com/connorjlink/geoRay Tracing
By simulating light rays with physically based materials, raytracer produces photorealistic scene renders with reflections, soft shadows, and global illumination.
github.com/connorjlink/raytracerRasterization
Powered by a custom linear algebra library, rasterizer features frustum culling, depth buffering, and face-based shading to view arbitrary .obj models.
github.com/connorjlink/constexpr-linear-algebra-library
Depicted below are sample renders from all three programs.
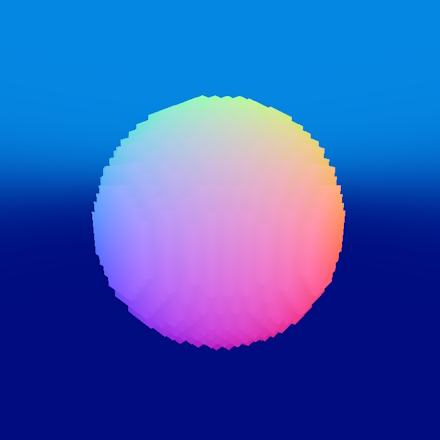
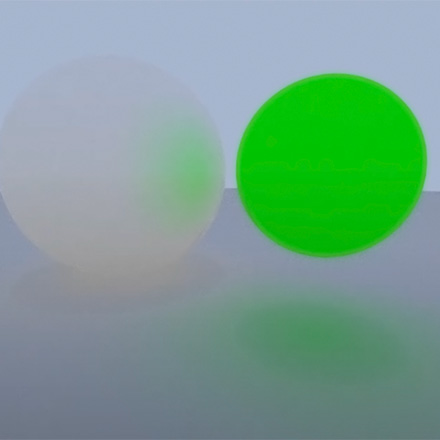
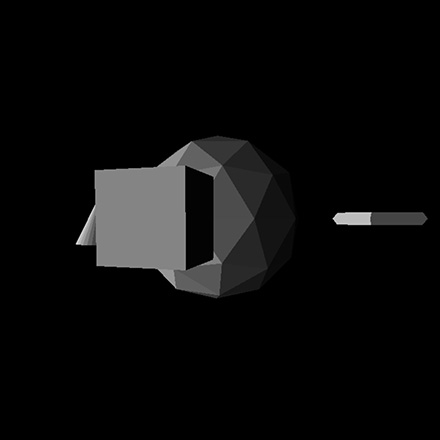
CL8
An entirely custom software suite for my 8-bit processor architecture, CL8.
Titanium
A novel programming language compiler designed for CL8, Titanium uses the GNU Flex/Bison lexer and parser generators to transform custom source code into flat CL8 assembly.
github.com/connorjlink/compilerDisplayed below are a sample program compilation input and output.
.macro BIT = (a):
{
(1 << [a])
}
function byte val test =
(byte val a,
byte val b,
byte val c)
{
return (a + b) | c;
}
function byte val main = ()
{
return $BIT(2);
}
.begin
.include "def.s"
@function_start_test:
ldb r0, %0
ldb r1, %1
adc r0, r1
ldb r1, %2
or r0, r1
push r0
@function_end_test:
pop IP
@function_start_main:
ldb r0, #1
rol r0, #2
push r0
@function_end_main:
pop IP
.end
Microcode
Extensively utilizing the C++ preprocessor, microcode synthesizes and serializes the custom μop sequences powering CL8 instructions.
github.com/connorjlink/microcodeAssembler
Based around a GNU Flex/Bison lexer and parser, assembler flattens custom assembly language source code into raw CL8 machine.
github.com/connorjlink/assemblerDisassembler
A fully custom binary analysis program, disassembler expands CL8 machine code back into human-readable assembly source for debugging.
github.com/connorjlink/disassemblerEmulator
Designed to painlessly debug CL8 binaries, emulator offers a readable behavior-level simulation of the CL8 architecture.
github.com/connorjlink/emulatorHardware
A register-transfer-level design, hardware implements the CL8 architecture in SystemVerilog for ultra-precise simulation.
github.com/connorjlink/hardware